Part 3: Developing with K2 connect
Part 3 moves into developing with K2 connect and how we might build our own Service Objects and SmartObjects from scratch. We will add a new service to call extended job data, then manually add custom methods. We will define our own parameters and the properties we want returned. We will define our own custom structure. To complete the manual process, we will map the new input and outgoing structure and properties to their corresponding BAPI input and outgoing properties.
We will add an additional service to call company data. Finally, we will update an existing service by adding an XML method to call the employee's contact email addresses. (In this case, the XML method allows us to call and return multiple properties back to one outgoing property.)
After defining new services and updating an existing service, we will manually create a SmartObject based on all three service calls. The SmartObject will list employees based on their last name. The extended job data is bound to the employee 'list' data by the employee's ID. The job data provides us with a company code, which in turn, becomes the bind to the extended company information.
What we specifically want to demonstrate in Part 3 is the ability to pull together a data set of information (employee > job > company) from multiple service calls, all in one SmartObject. We will then leverage that data from within our user interface, a SmartForm.

In this step, we are going to add a new project to Visual Studio for the Part 3 exercises.
Step 1 Tasks
- Create a new K2 Empty Project in Visual Studio and name it K2connectDev.
- Launch the K2 Service Object Designer. Expand the explorer nodes until you reach the K2LearningSAP node. Expand this node as well and when prompted, do one of the following:
- If you completed only Part 1 and have the login credentials embedded into the destination connection string, CHECK the option to use Predefined User Credentials.
- If you completed Part 1 and Part 2 and have enabled K2 SSO for K2LearningSAP, enter the following:
- UserName: k2learning
Password: K2pass!
- UserName: k2learning
- Define a Filter for the Rfc / Bapi Explorer with the following settings:
Filter Name: Employee BAPIs
Function: BAPI_EMP*
(Group should remain blank) - Locate the BAPI_EMPLOYEE_GETLIST method and test the connection using the K2 connect Test Cockpit. Use the following parameters to filter your results:
DATE(): CHECKED. Use the current date as the value.
LASTNAME_M: B*

- Launch Visual Studio if it is not already open. (Start > scroll to V> Visual Studio 2015)
- Create a new K2 Empty Project and name it
K2connectDev - Launch the K2 Service Object Explorer (View [ribbon tab] > K2 Service Object Explorer)
- In the explorer, expand the ERP Connect node, then the SAP R4.7 node. Expand the K2LearningSAP node and when prompted for credentials, use one of the following methods:
- If you completed only Part 1 and have the SAP credentials embedded in the destination connection string, CHECK the option to use Predefined User Credentials.
- If you completed Part 1 and Part 2 and have enabled K2 SSO for K2LearningSAP, enter the following credentials into the prompt:
- User Name: K2Learning
- Password: K2pass!
- Right-click the Rfc / Bapi Explorer title and select Add Filter. For the Filter Name, enter
Employee BAPIs
then for the Function, enter
BAPI_EMP*
then leave the Group value blank. Here, we are simply saying to filter out any method that does not begin with BAPI_EMP. - Expand the Rfc / Explorer node, then the Employee BAPIs node. (This is the filter we just added.) Locate the BAPI_EMPLOYEE_GETDATA method. Right-click the method and launch the Test Cockpit.
- When the K2 connect Test Cockpit opens, click Load Interface to expose the Input (parameters) and the Output (results) panes. Clear All of the Input parameters. CHECK the box next to the DATE() parameter and confirm the default value is the current date. CHECK the box next to the LASTNAME_M parameter and for the value, enter
B*
then Execute the test. - After a few seconds, click the PERSONAL_DATA > Show Details... return in the Output pane. If you see a table with results returned, your test is successful! (You can collapse the Input pane if you like to provide more room for the table.) Close the K2 connect Test Cockpit and return to the K2 Service Object Designer in Visual Studio.
In the next step, we are going to add a filter to the SAP methods returned so that it is easier to find what we need. We don't have to add filters, but when we have very large systems such as SAP, filtering our results can make our application development a little smoother.
Next, we will navigate to the method we need for our exercises, then test the method with the K2 connect Test Cockpit.
Step 1 Review
In this step, we added a filter to limit the methods returned by our sample SAP system. When developing with very large systems such as SAP, adding filters can make application progress much smoother. After adding the filter, we tested the SAP destination connection using the K2 connect Test Cockpit and confirmed we had results returned based upon the input parameters we entered.

In this step, we will add a new service method that will return a list of employees based on the employee's last name. We will select a specific set of properties that we want returned. We will also give each property a friendly name, so that the person who is designing the SmartObject will be able to tell exactly what the property is for by the friendly name. We will then add a second service method that will read one employee's details based on their employee ID. Last, we will disable the option to automatically create a SmartObject (from this Service Object) as we will manually create the SmartObject in the next step.
Step 2 Tasks
- Add a new folder to the K2connectDev project and name it ServiceObjects.
- Add a new K2 connect Service Object to the ServiceObjects folder and name it EmployeeDataServiceObject.
- Add a new Service and change the name to EmployeeData.
- Add the BAPI_EMPLOYEE_GETDATA method to the EmployeeData service.
- Change the Function name of the GETDATA method to ListEmpByLastName.
- Clear the pre-selected properties, then select the following properties and change their Friendly names as indicated:
Property New Friendly Name DATE() InputDate LASTNAME_M InputLastName PERSONAL_DATA EmployeeInfo RETURN() <No change> - Access the Structure Declaration for the EmployeeInfo property. Once again, clear the pre-selected properties, then select the following properties and change their Friendly names as indicated:
Property New Friendly name BIRTHDATE BirthDate BIRTHPLACE BirthPlace BIRTHYEAR BirthYear FIRSTNAME
FirstName FROM_DATE EmploymentDate LANGU Language LAST_NAME LastName NATIONAL Nationality PERNO EmployeeNumber - Return to the K2 Service Object Designer canvas and Save the project.
- Add another instance of the BAPI_EMPLOYEE_GETDATA method to the service. Change the Function name to ReadEmpByEmpID then Clear the pre-selected properties.
- Select the following properties from the Function Interface and give them friendly names as indicated in the table below:
Property New Friendly Name DATE() InputDate EMPLOYEE_ID InputEmployeeID PERSONAL_DATA EmployeeInfo RETURN() <No change> - There are no changes to the EmployeeInfo structure, so go back to the service design canvas.
- Disable the option to generate a SmartObject, then publish the new Service Object to the K2 Server.
- In the SmartObject Service Tester, confirm the new service object is shown under the K2 connect Service node. Take a minute to compare the methods, properties and parameters with the configurations we just made in Visual Studio. In particular, notice how the friendly names appear and why they might be easier to work with versus the system-generated property names.
The first method we will add will be the list method that returns a list of employee's based on the employee's last name.
The second method we will add will be the read method that will return a specific employee's details based on their employee ID.

- First we'll add a new folder to store the Service Object in. In the Visual Studio Solution Explorer, right-click the K2connectDev project and add a new Folder. Name the folder
ServiceObjects - Next, we'll add the new Service Object. Right-click the new ServiceObjects folder and Add a New Item. Confirm that the K2 Installed templates is highlighted, then select the K2 connect Service Object from the templates. Name the new Service Object
EmployeeDataServiceObjectThere are a number of limitations on the naming of Service Objects. For example, you cannot use a number to start the name or include periods in the name. If necessary, use underscores to separate words. - Click the Add Service link located in the upper right corner of the design canvas. The Service1 service is added. (See the image in step (e) for link location.)
- Double-click the Service1 title bar and change the name to
EmployeeData
then press <ENTER> to commit the change. - Expand the Employee BAPIs node, then navigate to the BAPI_EMPLOYEE_GETDATA method. Drag, then drop, the method into the EmployeeData container.
- Click the BAPI_EMPLOYEE_GETDATA method name to open its properties. Rename the method to
ListEmpByLastName
then Clear All of the listed properties. We will select a few properties to be returned instead. - Select the following properties:
DATE() This is the input date required for most SAP BAPIs. LASTNAME_M This is the input parameter to search by the last name. PERSONAL_DATA This is the structure of personal data returned by the BAPI. This property contains additional properties as well. RETURN() This is the return data (success message) returned by the BAPI. - Click on the DATE() value in the Friendly name column. You may need to click once or twice slowly to expose the text box. Change the name to
InputDate
then press <ENTER> to commit it.We are adding the 'Input' prefix to two of our property names so that the SmartObject designer can easily tell what the properties are used for. - Using the same actions as the step above, change the friendly names for the following:
LASTNAME_M: InputLastName
PERSONAL_DATA: EmployeeInfo - Click EmployeeInfo to highlight the row. Click the Show Structure link found in the upper right corner of the Function Interface pane. On the Structure Declaration pane, select Clear All to remove the pre-selected properties.
- In the Structure Declaration pane, CHECK the following properties and rename their Friendly names:
Property New Friendly name BIRTHDATE BirthDate BIRTHPLACE BirthPlace BIRTHYEAR BirthYear FIRSTNAME
FirstName FROM_DATE EmploymentDate LANGU Language LAST_NAME LastName NATIONAL Nationality PERNO EmployeeNumber - Click Go Back to return to the K2 Service Object Designer canvas.
- Save the project.
- Drag another instance of the BAPI_EMPLOYEE_GETDATA method into the EmployeeInfo service, just under the List EmpByLast Name method.
- Open the method properties and change the Function name to
ReadEmpByEmpID
then Clear All of the pre-selected properties. - In the Function Interface pane, use the values below to select the following properties, then change their Friendly names as indicated:
Property New Friendly Name DATE() InputDate EMPLOYEE_ID InputEmployeeID PERSONAL_DATA EmployeeInfo RETURN() <No change> - Access the structure for the EmployeeInfo (PERSONAL_DATA) property. The structure properties that we configured for the EmployeeInfo property should still be in place. You should see the specific properties we selected previously and their friendly names as well. There are no changes for this screen, so click the Go Back link to return to the K2 Service Object Design canvas.
- Save your project.
- Click the K2 blackpearl Settings icon found in the (left-column) explorer toolbar. UNCHECK the Generate SmartObject option. Confirm the Use Properties as Parameters option is also UNCHECKED.
- Publish the Service Object to the K2 connect server. Close the confirmation dialogue that indicates the ServiceInstance has been refreshed. Keep Visual Studio open.
- Launch the SmartObject Service Tester utility if it is not already open. (C:\Program Files (x86)\K2 blackpearl\Bin\SmartObject Service Tester.exe)
- Expand the ServiceObject Explorer > K2 connect Service > Connect Service for dlx (your server name may be different) > Connect Object nodes. Confirm that the EmployeeDataServiceObject we just published is there. (If you completed Part 2, you may also see the K2connectEmpData Service Object that was published as part of those steps.) You may need to refresh the Service Objects if you do not see the new service object.
- Review the methods, properties and parameters for the new Service Object. Notice that we have two methods (list and read). Review the parameters for the methods. Note that the parameters were automatically prefixed with p_ by K2.
In the next steps, we will add a service to the Service Object, then add the BAPI method that will retrieve a list of employees by their last name.
Next we will change the service name so that it's more meaningful, then we will configure the method properties we want returned when the call is made. We can also adjust the 'friendly names' for the properties which will come into play when we create and configure the SmartObject that will be based on this Service Object.
Next we will rename the parameters and properties so that they have 'friendly' names. This is especially useful to the SmartObject designer so that they can clearly distinguish one property from another.
The PERSONAL_DATA (EmployeeInfo) property has a structure which contains additional properties. We will now use actions similar to above and select specific properties for the structure, then give them friendly names as well.
Now we will add the READ method that will return one employee's details based on their employee number.
The last step we need to make is to disable the auto-generation of SmartObjects. We want to build our SmartObject from scratch, so we don't need K2 to create one for us when we publish our EmployeeDataServiceObject service object.
At this point, we will take a minute to confirm our Service Object appears in the SmartObject Service Tester. We should be able to see the properties and methods that have been returned from our SAP destination.
Notice the properties show the friendly names that we entered. Building the SmartObject will much easier with names that describe the property instead of the system-generated names. The EmployeInfo prefix is the friendly name we gave to the PERSONAL_DATA structure.
Step 2 Review
In this step, we added a new service method that will return a list of employees based on their last name. We modified the properties that will be returned and gave each property a friendly name. We then added a second service method that will read a specific employee's details by employee ID. We configured friendly names for this method as well. We disabled the option to automatically generate a SmartObject because we will build our SmartObject from scratch in the next step.
After publishing the new Service Object, we observed its properties, methods and parameters using the SmartObject Service Tester. In particular, the friendly names that provide a user-friendly scope to work with when we build the new SmartObject.

Now that we have our Service Object in place and have configured the methods and properties we need, we can build the SmartObject. The SmartObject will allow us to leverage the properties and methods returned from the SAP destination, in K2 Workflows and SmartForms. We will add both the list and read methods, then test the SmartObject functionality using the SmartObject Service Tester.
Step 3 Tasks
- In Visual Studio, add a new K2 SmartObject. For the Name, enter SAPEmployees.
- Switch the SmartObject Designer to Advanced Mode and remove all of the default SmartBox methods.
- Add a new method and name it ListEmpByLastName then set the Type to List and the Transaction to Continue.
- Add the ListEmpByLastName Service Object Method from the EmployeeDataServiceObject.
- Create All of the properties.
- Add another SmartObject method and name it ReadEmpByEmpID.
then make set the Type to Read and the Transaction to Continue. - Add the ReadEmpByEmpID Service Object method, again found in the EmployeeDataServiceObject.
- Auto-map the properties.
- Assign the p_InputDate Input Parameter to a new Parameter called p_InputDate.
- Assign the p_InputEmployeeID Input Parameter to a new Parameter called p_InputEmployeeID.
- Build and Deploy the solution.
- Test the new SmartObject with the SmartObject Service Tester by Executing the SAPEmployees SmartObject. Use the following values for the input parameters:
Method: ListEmpByLastName
p-InputDate: Current date
p_InputLastName: B*

- In Visual Studio, add a new folder to the K2connectDev project and name it
SmartObjects - Right-click the SmartObjects folder, then select Add, then New Item. Select SmartObject. (If you don't see the Installed K2 templates, search for K2.) Name the new SmartObject
SAPEmployees - Change the designer to the Advanced mode.
- In the SmartObject Methods pane, click the Remove All option, then OK when the confirmation dialogue appears.
- Add a new SmartObject method. (Click Next on the wizard landing page.)
- On the Method Details screen, add the following values:
Name: ListEmpByLastName
Type: List
Transaction: Continue
then click Next. - On the Add Service Object Method screen, browse for, and Add, the ListEmpByLastName method found in the EmployeeDataServiceObject. Use the image below as a guide to navigating to this method. (Keep in mind your dlx server name may be different.)
- Use the Create All button to auto-create the properties in the new SmartObject method. Finish the wizard.
- Save the SmartObject.
- Add a new SmartObject method with the following values:
Name: ReadEmpByEmpID
Type: Read
Transaction: Continue - On the Add Service Object method screen, browse to and Add the ReadEmpByEmpID Service Object method. (Keep in mind your dlx server name may be different.)
- Use the Auto-Map button to map the existing properties to the new Read SmartObject method.
- Click on the p_InputDate Input Parameter to highlight it. Click Assign. On the Map Service Method Parameter pop-up, change the Map To value to Parameter, then click the Create button.
- For the Parameter Name, enter
p_InputDate
then click OK twice to commit the change.You may see a default Parameter Name value of p_InputDate(1). This is because the SAPEmployees SmartObject has already registered a parameter named p_InputDate for the 'list' method. Parameters are bound to their associated methods, so it is safe to have the same parameter name for both the 'list' and 'read' methods. - Click the p_InputEmployeeID parameter to highlight it, then click Assign.
- Change the Map To value to Parameter, then click Create.
- For the Parameter Name, enter (or confirm)
p_InputEmployeeID
as the name value. Click OK twice to commit the change. - Finish the wizard. Save your SmartObject.
- Click the Build tab in the Visual Studio ribbon. Click Build Solution. When you see the Build Succeeded notation in the Status Bar (lower left corner of your screen), return to the Build menu and click Deploy Solution. Click Next twice on the Deploy Project Wizard, then Finish. Confirm you see a Deploy succeeded notation in the Status Bar.
- Launch the SmartObject Service Tester if it is not already open. Click Refresh All in the toolbar so that we see any changes that have been made since our last test.
- Expand the SmartObject Explorer and navigate to the SAPEmployees SmartObject using the image below as a guide. Take a minute to expand the Properties and Methods for this new SmartObject. Expand the Methods further and explore the Parameters for each.
- Right-click the SAPEmployees SmartObject title and select Execute SmartObject.
- CHECK the box for the p_InputDate Input parameter and confirm the default value is the current date. For the p_InputLastName value, enter
B*
then click Execute. Confirm you have results returns in the Results pane.
The SmartObject Designer opens. The SmartObject Designer is in Simple Mode by default. Notice the SmartObject Properties pane and the SmartObject Methods pane. The Methods pane contains default SmartBox Service methods. Because we have our own methods as part of our SmartObject (list and read), we will remove the default methods first.
Notice the Input Parameter, Input Property and Return Property values. This is another good example of why we use friendly names.
Using the similar steps as above, we will now add the read method.
In the next step, we will auto-map the existing SmartObject properties to the new 'read' method. We don't need to create the properties because we created them while setting up the 'list' method. To recap, the SAPEmployees SmartObject has a set of properties that we created while configuring the 'list' method. Since they are already set up, we can just map the 'read' properties to the existing SmartObject properties.
Now we will assign an Input Parameter bind for the p_InputEmployeeID parameter.
Your screen should look like the image below.
In Step 4, we will use our new SmartObject in a SmartForm. Before we can do that however, we need to build, then deploy, the SmartObject to the K2 server.
One last step we want to take before turning our attention to SmartForms is to test the new SmartObject using the SmartObject Service Tester.
Step 3 Review
In this step, we manually created a new SmartObject in Visual Studio. We added the two Service Object methods that were created previously, one returning a list of SAP employees based on the employee's last name. The other method will return the details of a specific employee based on the employee ID. After deploying the new SmartObject to the K2 Server, we tested it with the SmartObject Service Tester. We entered two input parameters (the input date and a wildcard input for the last name) then confirmed we had results returned.
What is especially significant is that we now have a SmartObject that can leverage the properties and methods of a SAP destination. This SmartObject can be called in K2 Workflows and SmartForms. In fact, in the next step, we will build a SmartForm using our SAPEmployees SmartObject.

If time permits, we can create a List View SmartForm using the K2 Designer. We will add two controls; a text box for inputting the last name and a button for firing off the SmartObject 'list' method call. We will then test our View and observe the results returned.
Step 4 Tasks
- In K2 Designer, locate the K2connectDev category that we created in Visual Studio. Add a new Category and name it
SmartObjects - Design a View from the SAPEmployees SmartObject using the following General settings:
Name: SearchEmpByLastName
Category: Views
View Type: List View
Data Source: SAPEmployees
List method: ListEmpByLastName
Call this method when the form loads: UNCHECKED - Create Labels and Controls and select the following Field Names to include in the list: EmployeeNumber, EmploymentDate, LastName, FirstName, BirthDate
- Change each Header Text value so that it reflects a friendly name. For our sample, we just removed the EmployeeInfo_ prefix and left the remaining common name.
- Add a Layout Table to just above the list layout table. Configure it to have 2 columns and 2 rows.
- Add a Label control to the first row, first cell. Change its Text value to Enter search string.
then add a Text Box control to the first row, second cell. Change its Name value to LastName Text Box then add a Button control to the second row, first cell. Change its Name to Search Button and its Text to Search. - Add a Rule to the View to that executes the ListEmpByLastName method when the Search Button is clicked. For the Input Parameters, use the System Date and the View's LastName Text Box control.
- Test the View using the Runtime URL. Enter a wildcard parameter for the search criteria (for example, B*) and confirm you have results returned that match the search input.

- Launch K2 Designer. (Start > scroll to K > K2 > K2 Designer)
- On the K2 Designer landing page, click BROWSE to open the explorer, or category browser.
- Expand the All Items node and locate the SAPEmployees SmartObject located in the K2connectDev category. Use the image below as a guide.
- Right-click the K2connectDev category and select New Category. Name the new category
Views
Next, we will design our new view. - Right-click the SAPEmployees SmartObject and select Design View.
- On the GENERAL screen, enter or select the following values:
Name: SearchEmpByLastName
Category: Views (Use the ellipses to select the Views category.)
View Type: List View
Data Source: Should be set on the SAPEmployees SmartObject
List method: Confirm the default is ListEmpByLastName
Call this method when the form loads: UNCHECKEDCall this method when the form loads. Because you designed the list view directly from a SmartObject, K2 by default will retrieve all content from the SmartObject when the view loads. In some cases, this may be the desired view behavior. If you need to filter the list content before it loads, uncheck this option. You can configure a rule that will load specific content. Examples include: if you want to load all contact records when the view launches, check this option. If you want to load only contacts from a specific department, uncheck this option and configure a rule to filter the records by department before they load. - Select the Create Labels and Controls option.
- On the Create Labels and Controls screen, select the following field to be included on the view: EmployeeNumber, EmploymentDate, LastName, FirstName, BirthDate (Depending on your environment, it may be difficult to see the entire field name. In that case, just check the first five options.) Do not make any other changes on this screen. Click OK to continue.
- On the Layout screen, enter a 'friendly' name for each of the column headings. First, highlight the column being edited. Then, in the Properties Pane, switch to the Header tab. Remove the Employee Info_ prefix from the Text property value. Repeat this for each of the column headings.
If you are new to K2 Designer, this is a typical representation of the list view layout. You are seeing a table format that at runtime, will be replaced by the actual values that are being returned for the list. From here, we can add controls at either the top or bottom of our table simply by dragging and dropping them from the Context Browser.
- On the left side of your screen, click the Toolbox tab. Locate the Layout > Table control. Drag it onto the design canvas, dropping it just above the list layout table. Set it to have 2 columns and 2 rows.
- Drag a Label control into the first row, first cell. Change the Text value for the label to
Enter search string
then drag a Text Box control into the first row, second cell. Change the Text Box Name to
LastName Text Box - Drag a Button control into the second row, first cell. Change the Name to Search Button
then change the Text to
Search - Events define when the rule should run, such as when a button is clicked, or when the form loads. (Events are optional, you can define "event-less" rules that you can call from other rules.)
- Conditions define whether the rule should run. If the criteria are true, continue the rule, and if the criteria are not met, stop the rule. For example, a condition might evaluate a form to confirm that required fields have content. (Conditions are optional - not all rules will require conditions.)
- Actions define what the rule should do. For example, show a message, start a workflow, or enable a form field. Rules can contain multiple actions that are run in sequence or in parallel.
- Click the RULES tab in the breadcrumb bar. Click Add Rule to get started. Confirm the Events tab is active, then locate (and click) the When a control on the View raises an event option to add it to the Rule Definition pane. (It's located under the Control Events heading.)
- In the Rule Definition pane, click the select control link and select the Search Button. The 'select event' configuration will be automatic because buttons pretty much just have one action and that's to be clicked!
- Click the Action tab so that it is active. This time, we will search for the Action we want to add. As you become more familiar with Rules and Rules terminology, you can speed up the process by searching keywords. In the Search box, enter
execute
then press <ENTER>. - Click the Execute a method on the View action to add it to the rule definition pane.
- Click the select method link and select the ListEmpByLastName method. Click the (configure) link. On the Input Mappings screen, notice the two Parameters that we previously created. Parameters are required values, so we must map them to the appropriate form and system values. Map the p_InputDate to the System Value > Current Date found in the Context Browser. Expand the SearchEmpByLastName View node, then the Controls node. Map the p_InputLastName to the LastName Text Box.
- Click NEXT, then FINISH the wizard. Click OK to complete the Rule Designer. Click FINISH to complete the View Designer. (If you are new to K2 Designer, the 'FINISH' button is near the upper right corner of your screen.)
- We are returned to the Category Browser and Properties Pane. With the SearchEmpByLastName View highlighted in the Category Browser, click the Runtime URL found in the central pane.
- The View will open in a browser window. Depending on your environment, it may take several seconds for the View to render. First, we want to enter our search criteria. In the text box, enter the following
B*
then click the Search button. After a few seconds, you should see results returned where every last name begins with 'B'.
Notice that the SAPEmployees SmartObject is located in the SmartObjects folder. This is the same folder we created for our Visual Studio project. K2 refers to the folders as 'Categories'. To keep things neat and tidy, we are going to create a new category to store our new view.
In this next step, we will add another layout table to the design canvas that will contain a text box control and a button control. The text box will be used for entering a 'last name' search parameter and the button control will call the SmartObject 'list' method when clicked.
The next step is to configure the rules that will fire when the Search button is clicked. To break it down, when the Search button is clicked, we want K2 to take the value that was entered into the LastName Text Box and return all of the SAP employee records containing that value. Behind the scenes, the rule will look something like: "When the Search button is clicked, execute the ListEmpByLastName method". If you are new to the concepts of K2 Rules, a brief explanation on Events, Conditions and Actions is provided in the NoteBox below.
Now that we have configured our 'Event', we can move on to configuring our 'Action'. We don't need any Conditions for this exercise, so we can just skip that tab.
Now, we will configure the Action so that it fires our list method, using the LastName Text Box value as a filter.
Step 4 Review
In this step, we used a SmartObject within a user interface, namely a SmartForms View. What is significant about this step is that we were able to create a view with data coming from a SAP destination. As the view designer however, we did not actually need to know where the data was coming from, just that it was available for use in the form of a SmartObject. Nor, did we need to know anything about the underlying data source connection configuration that was created for this SmartObject. We exposed data from a SAP system, to a form on a web page without writing a single line of code. Not bad!

In Step 5, we will extend the EmployeeDataServiceObject (Service Object) with additional methods and properties. We will learn how to define custom structures for complex return properties and how to add other BAPIs to an existing Service Object. Finally, we will learn how to return a complex property such as an XML property.
Overview
In the previous exercise, we created a simple SAPEmployees SmartObject with two methods: List and Read. Suppose that the organization would like to get more detailed information about an employee with the "Read" method, for example their Job Title, the Company that they work for and the employee's email address(es).
As it happens, all of this information is available from various SAP BAPIs. Consider the diagram below. We will be using two different BAPIs in this exercise: BAPI_EMPLOYEE_GETDATA and BAPI_COMPANY_GETDETAIL.
We used BAPI_EMPLOYEE_GETDATA in the previous steps and specifically we returned some fields from the PERSONAL_DATA return structure in the employee read method. In this step, we will use two other return structures for the same BAPI: ORG_ASSIGNMENT, where we can obtain the employee's job title, position and the organization they work for along with the COMPANY_CODE, and COMMUNICATION structure, which is a collection of e-mail addresses for the user. Since this is a collection, we will return data from this structure as an XML property.
To retrieve data about the company that the employee works for, we will use the BAPI_COMPANYCODE_GETDETAIL BAPI. Note that the ORG_ASSIGNMENT structure of the BAPI_EMPLOYEE_GETDATA method has a COMP_CODE return property: this is the return property we will use to retrieve the company information from the BAPI_COMPANY_GETDETAIL call.
Because Step 5 is quite extensive, we will break it down into three sections:
- Step 5a: Create a new service then manually configure the parameters and structure
- Step 5b: Add a method to retrieve the company information
- Step 5c: Update an existing method to include the employee's contact emails as an XML property

In this step, we will add a new service to our project, then manually define the parameters that we need. Two of the parameters will be input parameters and the third parameter will be a structure. After setting up our parameters, we will map them to output data fields, which essentially 'binds' the input data fields to the output data fields.
Step 5a Tasks
- Using the K2 connect Test Cockpit, execute the BAPI_EMPLOYEE_GETDATA BAPI method. Use the following values for the input parameters:
DATE: Current Date
EMPLOYEE_ID: 1037
then review the contents of the ORG_ASSIGNMENT return structure.
NOTE: You may need to provide credentials if you are working with the Single Sign On configuration that was set up in Part 2. The SAP system credentials are:
Username: K2Learning
Password: K2pass! - In the EmployeeDataServiceObject, add a New Service and name it JobData.
- Add a Custom Method to the new service and name it ReadJobDataByEmpID.
- Add a Parameter. This will be the parameter we need for the input date. Configure the parameter with the following options:
Name: InputDate
Declarer: Flat
Datatype: DateTime
Direction: In - Add a second Parameter for the employee ID with the following options:
Name: InputEmpID
Declarer: Flat
Datatype: Int64
Direction: In - Add the BAPI_EMPLOYEE_GETDATA method to the Internal Functions pane.
- Back in the Function Interface pane, Add a Parameter that we will define as our structure parameter. Name the new parameter JobInfo then select <New> for the Declarer.
- Name the new structure JobInfoStructure then select BAPIP0001B as the template we want to copy from. (The copy from Template link is located in the Structure Definition pane.)
- After clearing all of the pre-selected properties, manually select the following properties for the new structure: COMP_CODE, JOBTXT, ORGTXT, POSTXT
- Give each new property a friendly name. Use the table below as a guide.
Property New Friendly Name COMP_CODE CompanyCode JOBTXT JobTitle ORGTXT Department POSTXT Position - Go back to the Function Interface screen. Change the JobInfo Declarer value to Structure. Change the DataType to JobInfoStructure. Change the Direction to Out.
- Open the Data Mapping screen. Map the InputDate input data field to the DATE() output data field.
- Map the InputEmpID input data field to the EMPLOYEE_ID output data field.
- Finally, map the ORG_ASSIGNMENT return structure to the JobInfo structure. (Both of these values are found near the bottom of the Mappings categories.)
- Go back to the K2 Service Object Designer and Save your project.
Next, we'll add and configure the parameters we need for our service call. In our case, we will add a date and an employee ID for our input parameters.
Now we will manually define the structure we need for the employee's job data. Recall that a structure contains a number of properties, which we will define as well.
Now we need to define the mappings so that K2 connect knows how to pass the manual parameters we defined into and out of the BAPI.

- Open the K2connectDev project in Visual Studio if it is not already open. Open the Solution Explorer, then open the EmployeeDataServiceObject Service Object.
- Use the K2 Service Object Designer treeview to navigate to the BAPI_EMPLOYEE_GETDATA BAPI. You may need to provide credentials if you are working with the Single Sign On configuration that was set up in Part 2. The SAP system credentials are:
Username: K2Learning
Password: K2pass! - Right-click the GETDATA method and test it with the K2 connect Test Cockpit. Load the interface, then enter the following parameters (only):
DATE: CHECKED - current date
EMPLOYEE_ID: 1037 - Execute the method, then review the contents of the ORG_ASSIGNMENT return structure.
- In the Service Object Design canvas, click Add Service. Rename the new service to
JobData
then press <ENTER> to commit the change. - Click the Add Custom Method link located just below the JobData service title. Change the Function Name to
ReadJobDataByEmpID - Click the Add Parameter link. Change or confirm the following parameter values:
Name: InputDate
Declarer: Flat
Datatype: DateTime
Direction: In - Click the Add Parameter link to add our employee ID input parameter. Change or confirm the following values:
Name: InputEmpID
Declarer: Flat
Datatype: Int64
Direction: In - Save your project.
- From the K2 Service Object Explorer treeview, drag, then drop, the BAPI_EMPLOYEE_GETDATA method into the Internal Functions pane.
- Click on Add Parameter (back in the Function Interface pane). Name the new parameter
JobInfo
then for the Declarer value, select <New>. - Name the new structure
JobInfoStructure
then click the Copy from Template option found in the Structure Definition pane. - In the Template drop-down list, select BAPIP0001B, then Clear All of the pre-selected properties. CHECK the following properties to include in our custom structure: COMP_CODE, JOBTXT, ORGTXT, POSTXT.
- Click OK to save the selections. (See image above for reference.)
- In the Structure Declaration pane, rename each property with a friendly name.
Property New Friendly Name COMP_CODE CompanyCode JOBTXT JobTitle ORGTXT Department POSTXT Position - Click Go Back to return to the Function Interface screen. Change the Declarer for the JobInfo parameter to Structure, then change the DataType to JobInfoStructure and finally, change the Direction to Out.
Let's break this down a bit. Very simply put, a structure returns multiple properties for a single parameter. Because there could potentially be multiple datatypes within a single structure, we essentially create our own custom datatype (JobInfoStructure) that includes the package of properties and their datatypes. The flow of data is where the Direction comes in. We have 'in-coming' (In) parameters that are coming into SAP as filtering criteria, then we have 'out-going' parameters (Out) that are returning content, based on the filters.
- Click the Data Mapping link to begin.
- Click, then drag the InputDate parameter over to the right side so that it is mapped to the DATE() property.
- Repeat the process once again for the InputEmpID parameter and map it to the EMPLOYEE_ID property.
- Scroll down to the last section in the left-side column. Locate the Ref node. Map the ORG_ASSIGNMENT structure to the JobInfo structure found in the last node on the right-side.
- Click Go Back, the Go Back once again. Save your project.
In the next few steps, we will add a new service method that will retrieve the employee's job data. To give you more insight into structures, we will manually define the method.
Specifically, we are going to retrieve the job data as a separate service call because we did not need the content as part of the EmployeeData service. If we don't need the content returned, then it doesn't make sense to include it in the service call.
Recall that we previously added the 'list' and 'read' methods by dragging them from the BAPI list of methods into the EmployeeData service container. This time, we will define a custom method, essentially building the method from scratch.
Next, we'll add and configure the parameters we need for our service call. In our case, we will add a date and an employee ID for our parameters.
This is the standard date parameter expected by many SAP BAPIs. We will map these input parameters to the BAPI's input parameters manually.
Now we will manually define the structure we need for the employee's job data. Recall that a structure contains a number of properties, which we will define as well.
Now we need to define the mappings so that K2 connect knows how to pass the manual parameters we defined into and out of the BAPI.
Data mapping links the 'input' data fields with the 'output' data fields or structures. The left side contains the data structure for the filter, or in-coming data value. The right side contains the structure for the BAPI, or out-going data value. We map the two together by connecting one side to the other.
Step 5a Review
This last step was a long complicated procedure, but there is a reason we wanted to show you this approach. First things first: for this particular BAPI, we could have dragged and dropped the BAPI into the Service and selected the parameters and properties like we did before, so strictly speaking this longer procedure wasn't necessary for this particular BAPI. However, there may be times when you want to call multiple BAPIs in the same method, when you want to write some code as a completely custom function, or you may want to write some code to transform a parameter from one type into another type. In those cases you will need to manually define custom methods, define the parameters, design the structures and complete the mappings. We want you to be familiar with this approach so you know how to define these manually.

In Step 5b, we will add another new service, then configure the method to return the company details for an employee. For this step, we will return to the simple drag and drop process for adding new methods.
Step 5b Tasks
- Start by creating a new filter in the Rfc / Bai Explorer with the following details:
Filter Name: Company BAPIs
Function: BAPI_COMP* - From the new filter, run the BAPI_COMPANYCODE_GETDETAIL method using the K2 connect Test Cockpit, then review the results in the COMPANYCODE_DETAILS table.
- Open the structure details for the COMPANYCODE_ADDRESS structure, then Clear All of the properties. We won't be using them in this exercise.
- Add a New Service and name it CompanyData.
- Add the BAPI_COMPANYCODE_GETDETAIL method to the new service.
- Change the Function Name to ReadCompDataByCompCode.
- Show the Structure for the COMPANYCODE_DETAIL structure.
- Rename the Function to CompanyData.
then select the properties below to be included in this structure. Give each property a friendly name as well.Property Friendly Name CITY City COMP_CODE Company COUNTRY Country - Return to the Function Interface, then give the following two entries a friendly name:
COMPANYCODE_DETAIL: CompanyData
COMPANYCODEID: InputCompanyCode - Return to the Service Object Designer, then Save your project.

To get us started, we will take a minute to locate and run the method we need to return company details.
- In the K2 Service Object Designer explorer, add a new filter under the Rfc / Bapi Explorer node. For the filter details, enter the following:
Filter Name: Company BAPIs
Function: BAPI_COMP* - Expand the new company filter and locate the BAPI_COMPANYCODE_GETDETAIL BAPI method. Run the method in the K2 connect Test Cockpit using 1000 for the Company Code and reviews the results returned for the COMPANYCODE_DETAILS table. We specifically will be working with the COMP_NAME, CITY AND COUNTRY properties.
- Add a new Service called
CompanyData
then press <ENTER> to commit the new name. Drag the BAPI_COMPANYCODE_GETDETAIL method into the service. - Change the Function name to
ReadCompDataByCompCode - In the Function Interface screen, click Clear All. Notice that the fields aren't un-checked, because they are either structures or required input parameters. We need to "step into" each BAPI structure and select the fields to return.
- Click on COMPANYCODE_ADDRESS and then Show Structure.
- Click Clear All to remove the address details. In the real world, you would most likely want to include address details, but for our sample, we will not be using any of the address details. Click Function Interface to return to the previous screen.
- Click the COMPANYCODE_DETAIL structure and select Show Structure.
- Rename the Function to
CompanyData - Clear All of the pre-selected properties, then select the following: CITY, COMP_NAME and COUNTRY.
- Use the table below as a guide to give each of the properties friendly names.
Property Friendly Name CITY City COMP_CODE Company COUNTRY Country - Click Function Interface to return to the landing page.
- Rename the COMPANYCODE_DETAIL structure to
CompanyData - Rename the COMPANYCODEID property to
InputCompanyCode - Click Go Back to return to the Service Object Designer, then Save your project.
Step 5b Review
In this step, we added an additional method to return company details for the employee. When we eventually publish this service object, this method will become a separate K2 Service Object Method. (In a later lab exercise, we will add this Service Object method to our existing Employee SmartObject Read operation.)
In the next part, we will add another method to return the employee's communication data as an XML property.

In this step, we will add a method to return an employee's communication details. We will return the email address as an XML property. When working with XML properties, we will define custom methods so that we can manage how the data is treated.
Step 5c Tasks
- Add a new custom method to the EmployeeData service object. Name the new function ReadEmpCommicationsasXML.
- Add two new input parameters (input date and input employeeID) using the table below as a guide for the values.
Name Declarer DataType Direction InputEmpID Flat Int64 In InputDate Flat DateTime In - Add the BAPI_EMPLOYEE_GETDATA BAPI to the Internal Functions.
- Add a third new parameter and name it
CommunicationXML
then generate a New Declarer. Name the function
CommunicationXMLStructure
then mark the structure as an XML Property. - For the Structure Definition, select the Copy from Template option. Change the Template to BAPIP0105B then select the following options (only): USERID, USERTYPE, USERID_LONG (We don't need friendly names for these.)
- Go Back to the Function Interface screen and change the Declarer to Collection and the Direction to Out. Change the Datatype to CommunicationXMLStructure.
- The next step is the map the input parameters. On the Function Interface screen, access Data Mappings. Map the InputDate to the DATE() input. Map the InputEmpID to the EMPLOYEE_ID input.
- Finally, map the COMMUNICATION Ref to the CommunicationXML (Out) parameter.

- On the K2 Service Object Designer canvas, locate the EmployeeData service, then select Add Custom Method.
- Name the new function
ReadEmpCommunicationsasXML - Click on Add Parameter to add two new input parameters for the input date and the employee's ID. Use the values in the table below as a guide.
Name Declarer DataType Direction InputEmpID Flat Int64 In InputDate Flat DateTime In - In the Rfc / Bapi Explorer expand the Employee BAPIs node, then drag the BAPI_EMPLOYEE_GETDATA BAPI into the Internal Functions pane.
- In the Function Interface, click Add Parameter. Name the new parameter
CommunicationXML
then select <New> for the Declarer. - Change the Structure Name to
CommunicationXMLStructure
then CHECK the option to mark this structure as an XML property. - In the Structure Definition pane, select the Copy from Template option.
- Change the Template menu to BAPIP0105B, then select the following properties: USERID, USERTYPE, USERID_LONG
- Click OK, then Go Back to the Function Interface screen. (We don't need to rename any of these properties.)
- Change the Declarer to Collection and the Direction to Out. Change the Datatype to CommunicationXMLStructure.
- Click the Data Mappings link.
- Using the same process as we did configuring the JobData service, map the InputDate input parameter to the DATE() input. Map the InputEmpID to the EMPLOYEE_ID input.
- Then map the COMMUNICATION node from the Ref node to the CommunicationXML - Out property. (Both of these located at/near the bottom of their parent nodes.
- Click Go Back twice to return to the K2 Service Object Designer. Confirm the new ReadEmpCommunicationsasXML method appears in the EmployeeData service.
- Save your project.
Now we need to define how the parameters are sent to and retrieved from the BAPI by configuring the data mapping.
Step 5c Review
In this step, we added another method to return the employee's COMMUNICATION info as an XML property. The XML option is useful when the structure returns multiple items, or when you want to manipulate the XML property yourself in code.
In this example, the employee could potentially have multiple e-mail addresses, so that's why are returning this property as a XML property.
When using XML properties you usually need to define custom functions so that you can control the XML property. If the XML contains a collection of items, you need to map it to a Collection declarer.

Now that we have extended the K2 connect Service Object with the additional details (Job info, Company info and the emails as an XML property), we will extend the existing SAPEmployees SmartObject and include these methods in the ReadEmpByEmpID method. (We won’t be adding the additional properties to the ListEmplByLastname method, since it is not necessary to return detailed info when listing employees in our scenario.)
When the new SmartObject has been published we will use the SmartObject Service Tester confirm the SmartObject works as expected.
Finally, if time permits and if you completed the optional Step 4 of Part 3, (using a SmartObject in a view) you can extend the existing SmartForm-based user interfaces and create a new view which includes the detailed employee data. This view will be opened when a user clicks an employee record in the SearchEmpByLastName list view.
As we did in Step 5, we will break Step 6 down into three sections:
- Step 6a: Update the existing Service Object and SmartObject to include the additional data that was added in Step 5
- Step 6b: Test the updated SmartObject
- Step 6c: Extend the user interface (SmartForms) to include a "Employee Details" View (Optional)
Part 1: Update the existing SmartObject to include additional data
Part 2: Test the updated SmartObject
Part 3: Add a new View to show the additional employee data, and extend the existing SearchEmpByLastName list view to open the details view when a row is clicked in the list of employees

In this step, we are going to edit the existing SAPEmployees SmartObject and add the new employee job and company methods that we created in Step 5. We will then create a new property in the SmartObject to receive data from the employee's communication XML property.
Step 6a Tasks
- In Visual Studio, Publish the EmployeeDataServiceObject Service Object.
- Use the SmartObject Service Tester to view and confirm the new methods and properties have been added to the EmployeeDataServiceObject.
- In Visual Studio, open the SAPEmployees SmartObject. Edit the ReadEmpByEmpID method in Advanced Mode.
- Make sure the method is a Read type.
- Add the ReadJobDataByEmpID of the EmployeeDataServiceObject and confiure the two input parameters (p_InputDate and p_InputEmpID) to the existing SmartObject parameters.
- Map the Return Properties to new SmartObject properties.
- Add the ReadCompDataByCompCode service object method and configure the Company Code input parameter to map to the SmartObject Property (JobInfo_CompanyCode).
- Map the Return Properties to new SmartObject properties.
- Add a new SmartObject Property named CommunicationXML then make the data Type a Memo.
- Edit the ReadEmpByEmpID SmartObject Method and add a call to the ReadEmpCommunicationsasXML service object method.
- Map the input parameters to the existing SmartObject Method parameters.
- Configure the method to return the CommunicationXML property to the new CommunicationXML property.
- Save and Deploy the updated SmartObject.
In the next few steps, we are going to edit the existing SmartObject and extend the 'read' method to include the additional methods that we added to look up the job information and the company information.
First up, we'll add and map the job information.
Now we will add the company information.
Next, we will add a new SmartObject property for the returned XML value.

- In Visual Studio, publish the EmployeeDataServiceObject.
- Click Yes to confirm you want to replace the existing Service Object.
- You should see a dialog that states the Service Instance was refreshed. Close the confirmation notice.
- Launch the SmartObject Service Tester utility. (C:\Program Files (x86)\K2 blackpearl\Bin\SmartObject Service Tester.exe)
- Expand the ServiceObject Explorer and navigate to our K2 connect Service Object. We want to locate the EmployeeDataServiceObject, then review the properties and methods it contains.
- Confirm the new properties and methods we added are shown.
One thing to note is that importance of friendly names. You should see now how much of a difference it makes for a person developing the SmartObject to be able to easily distinguish the names and purposes of the properties they are working with.
- Return to Visual Studio and open the SAPEmployees SmartObject.
- Click to highlight the ReadEmpByEmpID method, then click Edit.
- On the wizard landing page, CHECK the option to Run the wizard in Advanced Mode, then click Next.
- Change the method Type to Read and confirm the Transaction is set to Continue, then click Next.
- On the Configure Method Parameters (Optional) screen there are no changes, so just click Next.
- On the Service Object Methods screen, we will add the two new service objects we created previously. Click Add.
- Use the Context Browser to navigate to the ReadJobDataByEmpID method of the EmployeeDataServiceObject Service Object. Drag, then drop, the method into the Service Object Method text box.
- Click to highlight the p_InputDate input parameter. Click Assign. Map it to the existing p_InputDate Parameter for the SmartObject method.
- Repeat this step and Assign the p_InputEmpID to the existing p_InputEmpID Parameter for the SmartObject method.
- Click to highlight the JobInfo_CompanyCode Return property, then click Assign. Click Create. Confirm (or enter) the JobInfo_CompanyCode Property Name. Click OK twice to return to the Edit Service Object Method screen.
- Repeat these steps to create properties for the remaining Return Property values.
Return Property Name Bound to SmartObject Property JobInfo_JobTitle SAPEmployees.JobInfo_JobTitle JobInfo_Department SAPEmployees.JobInfo_Department JobInfo_Position SAPEmployees.JobInfo_Position - Click OK to save the new method.
- Begin by selecting Add to attach a new Service Object Method.
- Using the Context Browser, navigate to, then Add, the ReadCompDataByCompCode method to the Service Object Method text box.
- Just like before, we need to assign a property to the p_InputCompanyCode input parameter. Click to highlight the p_InputCompanyCode parameter, then click Assign. Change the Map To value to Property, then select the JobInfo_CompanyCode Name from the drop-down list.
- Repeat the step above and Assign each of the Return Properties to new SmartObject properties. Remember to Create a new property with the same name value as the Return Property. (Highlight return value > Assign > Create)
Return Property Name Bound to SmartObject Property CompanyData_Company SAPEmployees.CompanyData_CompanyName CompanyData_City SAPEmployees.CompanyData_City CompanyData_Country SAPEmployees.CompanyData_Country - Click OK to accept the property mappings. Click Next, then Finish to complete the SmartObject Method wizard.
- Save your project.
- In the SmartObject Properties pane, click Add.
- Create a new SmartObject Property called
CommunicationXML
then make its data Type a Memo. - Edit the ReadEmpByEmpID SmartObject Method.
- Click through the Next buttons until you get to the Service Object Methods screen. Click Assign, then use the Context Browser to navigate to and Add, the ReadEmpCommunicationsasXML method.
- Assign the p_InputDate input parameter to the existing SmartObject parameter with the same name. Assign the p_InputEmpID input parameter to the existing SmartObject parameter with the same name.
- Click to highlight the CommunicationXML Return Property. Click Assign. On the XML Property Mapping screen, click Add.
In the Add XML Property Mapping screen, select the connect node, then set the XML Mapping Type to Xml. Set the Map To value to Property. Select the CommunicationXML [Memo] property we created earlier from the Name drop-down list. Click OK twice to complete this mapping. - Back on the Edit Service Object Method screen, confirm the CommunicationXML property is mapped to the SAPEmployees SmartObject. Click OK, then Finish to complete setting up the method to return the XML property.
- Save and then Deploy the updated SAPEmployees SmartObject. When the deployment has completed successfully, you can close Visual Studio. We have completed all of Visual Studio steps for this tutorial.
We'll take a minute now to test the update Service Object and SmartObject using the SmartObject Service Tester.
Minimize the SmartObject Service Tester. We will use it again in a later step.
In the next few steps, we are going to edit the existing SmartObject and extend the 'read' method to include the additional methods that we added to look up the job information and the company information.
When using multiple BAPIs in a sequence (when those BAPIs need to transfer data between each other such as an employee ID) we need to expose each method as a separate Service Object, then 'chain' the methods together inside of the SmartObject.
Notice the Input Property and Return Property values load. We need to map the input parameter to the SmartObject method to establish a communication link between the two.
Now we need to create SmartObject properties for the Return values. We cannot map these to existing properties because they do not exist in the SAPEmployees SmartObject. We will create new properties instead.
We've just added the first of the additional methods required to read additional employee details. What is especially significant here is that we retrieved the employee's CompanyCode as part of this method, so now we can use that property to call the ReadCompDataByCompCode method and retrieve information about the company that the employee works for. We will use the CompanyCode as the input parameter for retrieving data from the company details method.
We can see now that the ReadEmpByEmpID method now calls three separate Service Object methods to build a collection of detailed employee information. This method can now retrieve employee personal details, the employee's job details and the employee's company details.
Our final step in building the SmartObject properties is to add a property to capture the returned XML employee communication data. Generally, we want to return XML properties as 'Memo' data types, but we can manually map XML properties to SmartObject properties as well.
Now we need to bind the CommunicationXML Return Property to the new SmartObject property 'Memo' property.
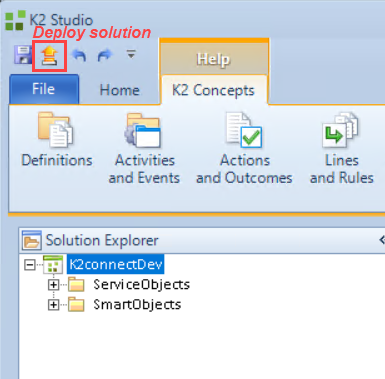
Step 6a Review
We have now updated the SAPEmployees SmartObject to call the additional methods that return more detailed information about the employee. Remember each method is a separate call to different SAP BAPIs, so this way, we can build up a composite SmartObject which returns data from different SAP BAPIs, passing in return properties from one method as the input parameters for a subsequent method.
Remember also that we could have added any other Service Method as an additional method, so you could have included data from any other system as part of the SAPEmployees detailed information. For this learning module, we are only using data from SAP, but the principle is the same: we can "chain" multiple service method calls in a single SmartObject method call to build up a SmartObject that combines data from different systems, (as long as we define the sequence of operations correctly and we pass values between method calls correctly).
We also added a new XML property to return a BAPI Structure as an XML property rather than "flattening" it to a collection of SmartObject properties.
In the step part we will test our updated SmartObject.

In this step, we will once again return to the SmartObject Service Tester to test our latest SmartObject configuration. After entering the input date and Employee ID parameters, we will confirm the data returned includes the employee's job information and company information.
Step 6b Tasks
- Use the SmartObject Service Tester to execute the ReadEmpByEmpID method found within the SAPEmployees SmartObject. Use the following input parameters, then confirm you have a result returned that shows the additional properties (job information and company information):
p_InputDate: Current date
p_Input EmployeeID: 1037

- Open the SmartObject Service Tester once again. Expand the SmartObject Explorer and navigate to the ReadEmpByEmpID method for the SAPEmployees SmartObject. Right-click the method and select Execute Method.
- Recall that we have two parameters that are required: the input date and the employee's ID. Locate the p_InputDate input parameter, then CHECK the box to the left of the date value, then confirm the date value reflects the current date. For the p_InputEmployeeID value, enter
1037
then click Execute. -
You should see a list of the additional properties (JobTitle, Department, Company and so on) along with an XML property containing the email records for the employee.
Step 6b Review
In this step, we returned to the SmartObject Service Tester and confirmed our additional methods (and XML property) have been configured properly. At this point, we are now ready to use the SmartObject within K2 Workflows and SmartForms.
The next step is optional if time allows, but it nicely demonstrates the use of our extended SmartObject in a SmartForm View.

You will only be able to complete this part of the tutorial if you completed the previous optional step to show a list of employees on a SmartForm View. In this step, we will create another View to show the employee details when the user clicks an employee's name from the list.
Step 6c Tasks
- In K2 Designer, Design a View from the SAPEmployees SmartObject with the following settings:
View Name: Employee Details View
Category: Views
View Type: Item View - Use the Create Label and Controls options to create a 2-column layout table with All Fields Included and Displayed.
- Located the Communication XML control and merge its table cell with the cell to the right.
- Generate a Form from the SearchEmpByLastName list View and name the Form Employee Data then Save and edit the Form. (Be sure to UNCHECK the option to Refresh the List View when the Form loads.)
- Add a Rule to the Form to open the Employee Details View when a row in the SearchEmpByLastName View is clicked. Configure the Rule Action to call the method which populates the Employee Details View, and pass in the EmployeeID of the selected row.
- Use the Runtime URL to test the SAP configuration. Enter a search string such as B*, then click one of the rows after data has been returned. Confirm the subview pops up with the extended employee details.

- Launch K2 Designer. (Start > scroll too K > K2 > K2 Designer)
- Click BROWSE on the landing page. Navigate to the K2connectDev node, then expand the SmartObjects folder. Right-click the SAPEmployees SmartObject, then select the option to Design View.
- On the General screen, Name the new View
Employee Details View
then change the Category to the Views category, then confirm the View Type is an Item View. The Data Source should already show the SAPEmployees SmartObject (remember, we designed the View from this SmartObject). Click CREATE. - Select the option to Create Labels and Controls.
- On the Create Labels and Controls settings screen, leave the default number of Columns at 2. Select all of the fields to Include on the View in Display Only mode. Leave the remaining settings as is and click OK.
- Locate the Communication XML control (it should be in the last row) and extend its table cell so that it is merged with the cell to the right. (Click once in the first cell, then click the Merge Cell to the Right icon found in the View Canvas menu bar.)
- To save time, we will not format the View's styling or layout. Click FINISH to complete the View build. The Finish button can be found new the lower right corner of the View design canvas.
- From the Category Browser, Right-click the SearchEmpByLastName View and select the option to Generate Forms.
- Change the Name of the Form to
Employee Data
then CHECK the option to Save and edit the Form. UNCHECK the option to Refresh the List View when the Form loads. Click OK to continue. - The Form will now open with the design canvas in the LAYOUT screen. We will now add the rules for populating the Employee Details View. Click the RULES tab found in the breadcrumb bar. On the Rules screen, click Add Rule.
- Events define when the rule should run, such as when a button is clicked, or when the form loads. (Events are optional, you can define "event-less" rules that you can call from other rules.)
- Conditions define whether the rule should run. If the criteria are true, continue the rule, and if the criteria are not met, stop the rule. For example, a condition might evaluate a form to confirm that required fields have content. (Conditions are optional - not all rules will require conditions.)
- Actions define what the rule should do. For example, show a message, start a workflow, or enable a form field. Rules can contain multiple actions that are run in sequence or in parallel.
- Confirm that the Events tab is active. Locate the When a View raises an event event and click it once to add it to the rule definition pane. Since we only have one View on this Form, the View name should already indicate the SearchEmpByLastName View. Click the select method link and select the List item click method.
- Now switch to the Actions tab. We've told K2 the event is when a list item on this view is clicked, now we need to tell K2 what to do after the list item is clicked. (In our case, we don't need a condition, but if you were to have conditions, you would add them prior to adding the action.)
- On the actions screen, locate the Open a subview and execute a method action (under the Subview Interaction heading) and click it once to add it to the rule definition pane.
- Click the select View link the, navigate to, and select, the Employees Details View. Click the select method link and select the ReadEmpByEmpID method that we previously created in Visual Studio.
- Now we need to map the input parameters that are required for this method. Recall that they consist of the p_InputDate and the p_InputEmployeeID properties. Click the (configure) link.
- Click the Input Mappings tab. Expand the Context Browser, then drag the System Values > Current Date into the p_InputDate Parameter.
- Now we need to add the employee ID parameter. We will find this value located in the Employee Data (Form) node, then SearchEmpByLastName (View), then SAPEmployees SmartObject, then Employee Info_Employee Number property. Drag this property into the p_InputEmployeeID Parameter.
- Click FINISH to complete the subview method. Click OK to complete the Rule configuration. Finally, click FINISH once again, to complete the Form build.
- From the Category Browser, click once on the Employee Data Form. Click the Runtime URL link found in the Properties pane.
- The Employee Data Form will open in your browser. Search for wildcard value
B* - When the list of employees is returned, click on one of the records. The subview should pop up with that details from the employee selected.
We have a few more steps to complete before we can test our expanded SmartForms configuration. Next, we will generate a Form from the SearchEmpByLastName list View. Then we will add a Rule for when a row in the list View is clicked. We will configure this Rule to populate the Employee Details View and pass the Employee ID from the selected row to the Details View.
This action is telling K2 to open another view as a subview (think pop-up window), then execute a method. In our case, the method will be to populate the subview (the Employee Details View) with the details of the employee that was selected from the list. We will configure the method to pass the employee's ID from the list item to the subview so that K2 knows which employee record to retrieve for the details view.
To close out this tutorial, we will test our extended configurations.
Step 6c Review
In this step, we tested the extended Service Object Method configurations. We generated an item View, then a Form. We configured Rules that will populate a subview (the Employee Details View) with the extended methods (job and company information) when a row is clicked in the list View.
What is significant here is that we are able to use multiple sources of data and return it to one location. While we are using data from an SAP system, we don't have to. We could "mix and match" data from multiple data source types and return them in one SmartObject.
Last, if you are new to SmartForms, this step gave you a nice introduction to Rules: Events and Actions and how you can configure them to provide additional information to your users in a subview format.